Hi All,
I am back with the series of BDC.
I suggest to go and read below articles on BDC before proceeding here.
Business Data Catalog (BDC) Part - 1Business Data Catalog (BDC) Part - 2Business Data Catalog (BDC) Part - 3Business Data Catalog (BDC) Part - 4Business Data Catalog (BDC) Part - 5and later
Business Data Catalog (BDC) Part - 7Let's continue the series and start our part 6.
Ok, in this article we are going to discuss about the relations between the tables. We have relations in our table with primary key and foreign key concept in database. Here relationship in terms of BDC web parts with the keys.
So let’s jump in to practical to understand it better.
We will use the Same XML that we used in previous part 5. Always remember to keep using latest XML that we keep modify in each part one by one.
Before proceeding further we will change our XML now to include au_id primary key in this XML. So that we can use it for relation with other tables through XML as well.
We will take authors and titleauthors. Which authors has written what titles and royaltyper column from it. It is enough to see the scenario in action although may not sound logical.
Ok, you need to add one more Entity Type in our XML in <Entities> Tab.
<Entity EstimatedInstanceCount="20" Name="Title">
<Identifiers>
<Identifier Name="title_id" TypeName="System.String"/>
</Identifiers>
</Entity>
Now that once we have added this.
Go inside <Entity EstimatedInstanceCount="100" Name="Author">, we have <Methods> tab, go inside that and paste this code.
<Method Name="GetTitlesForAuthors">
<Properties>
<Property Name="RdbCommandText" Type="System.String">Select au_id, title_id, royaltyper From titleauthor Where au_id=@au_id</Property>
<Property Name="RdbCommandType" Type="System.String">Text</Property>
</Properties>
<Parameters>
<Parameter Direction="In" Name="@au_id">
<TypeDescriptor TypeName="System.String" IdentifierName="au_id" Name="au_id"/>
</Parameter>
<Parameter Direction="Return" Name="Titles">
<TypeDescriptor TypeName="System.Data.IDataReader, System.Data, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" IsCollection="true" Name="SalesOrderDataReader">
<TypeDescriptors>
<TypeDescriptor TypeName="System.Data.IDataRecord, System.Data, Version=2.0.3600.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" Name="SalesOrderDataRecord">
<TypeDescriptors>
<TypeDescriptor TypeName="System.String" IdentifierEntityName="Title" IdentifierName="title_id" Name="title_id">
<LocalizedDisplayNames>
<LocalizedDisplayName LCID="1033">title_id</LocalizedDisplayName>
</LocalizedDisplayNames>
</TypeDescriptor>
<TypeDescriptor TypeName="System.Int32" Name="royaltyper">
<LocalizedDisplayNames>
<LocalizedDisplayName LCID="1033">royaltyper</LocalizedDisplayName>
</LocalizedDisplayNames>
</TypeDescriptor>
</TypeDescriptors>
</TypeDescriptor>
</TypeDescriptors>
</TypeDescriptor>
</Parameter >
</Parameters>
</Method >
Also add the association right after </Entities>
<Associations>
<Association AssociationMethodEntityName="Author" AssociationMethodName="GetTitlesForAuthors" AssociationMethodReturnParameterName="Titles" Name="AuthorToTitle" IsCached="true">
<SourceEntity Name="Author" />
<DestinationEntity Name="Title" />
</Association>
</Associations>
As you come to know here, what we did is we have used au_id as input paramter to the new destination, that is titleauthor table.
We want to display title_id and royaltyper columns in that new webpart which soon we are going to use on our page for the relation.
And finally then we have made the association between two entities for having parent child relationship.
Ok, now let’s go ahead and add one more web part on the page.
Edit the page, and add a web part named “Business Data Related List”.
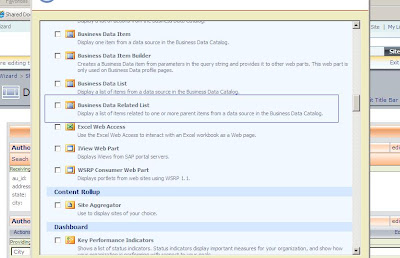
Now modify the newly added web part and select AuthorTraders in Type selector. The moment you do this, we can come to know the relationship that we defined automatically selected in relationship dropdown in terms of AuthorToTitle.
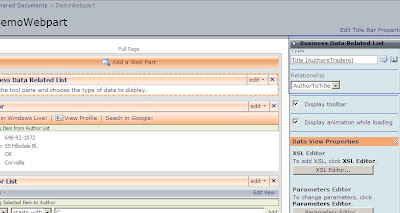
Once, you have that webpart on your page, next step is to connect that web part with the parent one. So go ahead to the Author List web part, the main parent web part and connect it with the TitleList.
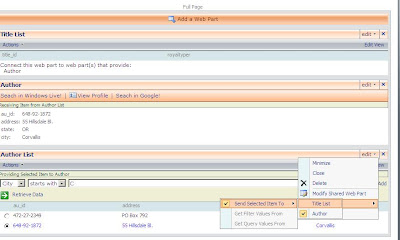
And see the magic. Select au_id from parent list and see both web parts will give you the result.
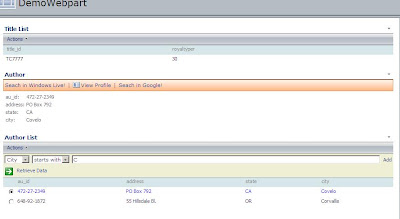
I will continue the series further for more detailing on BDC.